Python Programming Essentials
First a disclaimer. The this page is not a comprehensive introduction to programming or the Python language—it covers only ideas that are essential for using the ToolBox. If you want to really learn the ins and outs of Python in particular or programming in general, take a look a one of these sites:
- How to Think Like a Computer Scientist: Learning with Python
- An excellent introduction to programming using Python.
- The Python Tutorial
- This is the Python tutorial on the Python Programming Language website.
Variables and object types
Programs operate on objects. For example, we might assign an object to a variable x
with the assignment statement x = 45
. In this case, the object is a number, but in Python we could also assign a string of characters to a variable, s = 'spam'
. In this case the object's type is a string of characters. Before we delve into the details of object types, we should learn a few of the subtleties of variables.
A variable is just a name that refers to some object. In Python the assignment statement x = 45
allocates some space in memory for the variable x
and then stores the value 45 in it. In Python variable names can be arbitrarily long, they can contain letters and numbers, but they can't begin with a number. They are also case sensitive so N
and n
are different variables. You can also us the underscore characters '_
', but steer clear of other special characters; they have a specific meaning in Python. The current version of Python (3.5.1) also has 33 keywords that can't be used as variable names. The keywords have special meaning in Python. You're already familiar with a few of them, def
for example. Here's the complete list:
'False', 'None', 'True', 'and', 'as', 'assert', 'break', 'class', 'continue', 'def', 'del', 'elif', 'else', 'except', 'finally', 'for', 'from', 'global', 'if', 'import', 'in', 'is', 'lambda', 'nonlocal', 'not', 'or', 'pass', 'raise', 'return', 'try', 'while', 'with', 'yield'
Finally, don't confuse the assignment statement with with a mathematical equation. They may look the same, but the equals symbol in Python represents variable assignment not equality. For example x = x + 2 makes no sense mathematically, because if you try to solve it you get 0 = 2. However, the Python statement x = x + 2
is valid. It says assign the value of x
plus 2 to x
. If x
was 1 before the x = x + 2
statement it will be 3 after the statement. If you are confused by this, try it out in Python. It won't complain.
Numeric types
Scientists work with numbers, but there are several kinds of numbers: integers, real numbers, complex numbers, etc. Computers store each of these different numeric types in a different ways in memory. Computer languages like Java and C++ require that you declare an variables's type before you assign an object to it. Python determines the type from the assignment. The table below shows how a few of Python's numeric types are typed in a program and how they are interpreted.
What you type | Interpretation |
---|---|
n = 137 y = -99 m = 0 |
integers |
x = 3.14 r = 6.63e-34 y = 7.e23 |
floating-point (real numbers) |
x = 1.2+5.6j y = 3+4j z = 6.2-8J |
complex numbers |
You can use type()
to determine the type of any variable.
In [1]: myint = 123 In [2]: myfloat = 137.035 In [3]: mycomplex = 3+2j In [4]: type(myint) Out[4]: <type 'int'> In [5]: type(myfloat) Out[5]: <type 'float'> In [6]: type(mycomplex) Out[6]: <type 'complex'>
Exercise 1
Assign variables to two of each type of numeric object: integer, floating point number, and complex number. Multiply each of the following types by one another and fill out the table below.
The three numeric types discussed above are built-in to Python. There are others, but these are the most useful for our purposes. Python allows you to build your own objects types and define methods for operating on those objects, but most of the time we can get by with the built-in types and with a few extensions.
Strings
Another built-in type is a string. A string is an array of characters. Strings are used to represent any text-based information such as file names, and text printed out by your program. Strings are assigned to variables in the same way as numeric objects, but we must put the string text in single or double quotes. For example, str1 = 'green eggs'
and str2 = "ham"
are both acceptable ways of assigning strings to variables. You can even operate on strings. For example:
In [23]: str1 = 'green eggs' In [24]: str2 = "ham" In [25]: str3 = str1 + ' and ' + str2 In [26]: print(str3) green eggs and ham
For strings, the +
operator concatenates the operands.
Exercise 2
Experiment with numeric and string types by entering the commands listed below. Try to summarize the rule Python uses to give each result. In some cases Python may give you an error message. Try to understand why?
str1 = "egg's" |
str2 = 'egg"s' |
str = 'Ni!' |
s = '5' |
len(str1) |
st = "hello" |
longstring = 'The knights who say "Ni!"' |
Don't be afraid to experiment with your own commands. Once you think you understand how Python handles these commands, check your work on the Python Programming Essentials solutions page.
Python lists
Another Python built-in object type is a list. A list is just a sequence of objects. A list is declared by using square brackets. Here are a few examples.
In [3]: mylist1 = [1, 2, 3] In [4]: mylist2 = [1.34, 2.68, 9.83] In [5]: mylist3 = ['eggs', 'spam'] In [6]: mylist4 = [1, 3.14, 'eggs', mylist1] In [7]: print(mylist4) [1, 3.1400000000000001, 'eggs', [1, 2, 3]]
The first list, mylist1
, is an sequence of integers, mylist2
is an sequence of floats, and mylist3
an sequence of strings. The last list, mylist4
, is a mixed-type list. It consists of an integer, a float, a string, and a list! This last list may not be very useful, but it is allowed by Python. There is a lot to learn about Python lists and related objects called tuples, but we'll introduce new concepts as we need them in the Toolbox. Go to the Python Tutoral if you would like to learn more about lists or tuples right now.
Numpy arrays
We discussed PyLab briefly on the Quick Tour page. PyLab actually consists of a collection of packages called Numpy, SciPy, and matplotlib. All of these packages use an array object defined in the Numpy package. It would be nice if the PyLab package could use Python lists directly, but it can't. Numpy arrays can be made from Python lists, but there are many ways to create Numpy arrays. One is by using the linspace()
command you met on the Quick Tour page. The table below shows a few examples of ways to make Numpy arrays.
Command | Description |
---|---|
myary = array([2,3,4]) |
This created the Numpy array myary from the Python list [2, 3, 4] . |
x = linspace(1,100,1000) |
This creates x with 1000 elements evenly spaced from 1 to 100. |
Nutn = zeros(10) |
This creates Nutn with 10 elements, all zeros. |
SomeOnes = ones(10) |
An array of ten ones |
myrands = rand(10) |
An array of ten random numbers in the interval from 0 to 1 |
y = logspace(a,b,100) |
y is an array of 100 numbers logarithmically spaced from 10a to 10b. |
Open up a IPython session and try a few of these commands. Remember, Numpy arrays are part of the PyLab package so you have to import PyLab before you can use the commands.
Exercise 3
Create some Numpy arrays. Try adding, subtracting, multiplying, and dividing arrays by numbers and by other arrays. What is allowed and what isn't? What rules does the Numpy package use to determine the result? Once you think you understand how Python handles these commands, check your work on the Python Programming Essentials solutions page.
Keyboard input and output
Keyboard input
You can use the built-in command input(your_prompt)
to read strings from the keyboard where your_input
is any valid string.
In [5]: color = input('What is your favorite color? ') What is your favorite color? blue In [6]: print(color) blue
If you wish to input a numerical type, you can use import()
to get the string equivalent then convert it using one of the built in functions float(x_str)
, int(x_str)
, or complex(re_str,im_str)
. For example to read in a floating-point number:
In [7]: number_string = input('Type a number: ') Type a number: 5.0 In [8]: number = float(number_string) In [9]: print(number) 5.0
A more concise way to accomplish the same thing would be to combine the first two commands.
In [12]: number = float(input('Type a number: ')) Type a number: 7 In [13]: print(number) 7.0
Exercise 4
Download the input_test.py
program and run it with a few different types of input. Use the type()
command to examine the object type of the name
and x
variables after the program has run. What happens if you enter a non-numerical characters for the number? Once you think you understand how Python handles these commands, check your work on the Python Programming Essentials solutions page.
Formatted print
We have already used the print()
statement. We've used it to to display all sorts of Python objects. The print()
command gives you a great deal of control over the format of the output. The Exercise 2 and the input_test.py
program illustrates how to use print()
to format output.1 Consider the code from Example 2.
In [6]: st = "hello" In [7]: p = 3.14 In [8]: print("%s, the value of p is %g" % (st,p)) hello, the value of p is 3.14
The general syntax is print("string with format specifiers" % (variables))
. In this case the format specifiers are %s
and %g
. The variables are st
and p
. The format specifiers tell Python the object type for each variable. In this example st
is a string; the format specifier for a string is %s
. The variable p
is a float; %g
is one of a few different format specifiers for floating point numbers. The table below lists a few of the most useful format specifiers.
Format specifier | Type |
---|---|
%s |
string |
%d |
integer |
%f |
floating point numbers |
%e |
floating point numbers in scientific notation, the mantissa and exponent are always present |
%g |
floating point numbers, omitting trailing zeros and the decimal point if it's not needed |
You can also insert a tab or a new-line character into the output by typing \t
or \n
respectively. For example:
In [9]: x = 1.2 In [10]: y = 2.2 In [11]: z = 3.3 In [12]: print("x \t y \t z \t\n%g \t %g \t %g" % (x,y,z)) x y z 1.2 2.2 3.3
The function print()
is based on the C-command printf()
. It's a complex function with lots of parameters, but the ones listed above are the most useful. If you are working on a Unix-based computer (Mac or Linux) you can see a full description of the command by typing man printf
at the Unix command line.
Controlling the flow
if, elif, else
Sometimes you need your program to execute different code depending on some condition. The if
statement is probably the most useful of these flow-control statements. The if_test.py
program shows how the if statements work. Download and run it a few times. Try to predict the programs output for every input you try.
""" if_test.py Program to illustrate how if statements work. It uses input() to read strings from the keyboard then tests the input string with an if statement to determine the output. """ colour = input("What is your favorite color? ") warm_colours = ["red","yellow","orange"] cool_colours = ["blue", "green", "purple"] if (colour == "black"): print("Your favorite color is Black.") print(" That is an unusual favorite color.") elif (colour == "white"): print("Is white really a color?") elif (colour in warm_colours): print("You like warm colors.") elif (colour in cool_colours): print("You like cool colors.") else: print("Sorry, I don't know the color '%s'." % colour)
The syntax of the if
statement is:
if (conditional statement): block of code elif (conditional statement): block of code else (conditional statement): block of code
The elif
and else
parts of if statements are optional. A conditional statement is a programing statement that is evaluated as either True
or False
. For example colour=="black"
tests the string colour
and returns True
if the value of the string colour
is identical to "black"
. The equality test operator ==
can be used with almost any variable type. The table below lists some of the most useful comparison operators.
Operator | Read as |
---|---|
== |
is equal to |
!= |
is not equal to |
< |
is less than |
> |
is greater than |
<= |
is less than or equal to |
>= |
is greater than or equal to |
You can use and
, or
, and not
make compound conditional statements. You can learn more about how use these statements when you need them from the Python Language Reference.
INDENTATION IS IMPORTANT IN PYTHON!! The blocks of code after each conditional statement must be indented. The indentation tells the Python interpreter all the indented code after the conditional statement is to be executed.
Exercise 5
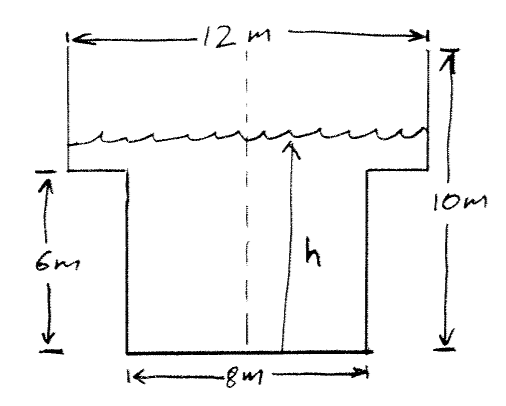
The cylindrical tank on a water tower has the cross-section shown in the figure above. Write a program that takes the height h of the water from the bottom of the tank as input and returns the volume of water in the tank, and tells you that you've made an error if h greater than the height of the tank.
The for
statement
The for statement allows you to repeat a block of commands. Here's an example program:
""" for_test.py Program to illustrate how for statements work. The program prints the x and y positions of a projectile launched at a 45 deg angle. """ vx = 50.0 vy = 50.0 g = 9.8 print(' x \t y ') for t in range(1,11): # range(1,11) creates the list [1,2,3,4,5,6,7,8,9,10] x = vx*t y = vy*t - 0.5*g*t**2 print('%g \t %g' % (x,y)) print('\n') # Here is another way to generate idential output using numpy # arrays from pylab import * t = linspace(1,10,10) # creates a numpy array x = vx*t y = vy*t - 0.5*g*t**2 print(' x \t y ') for idx in range(0,10): print('%g \t %g' % (x[idx], y[idx]))
The syntax of a for
statement is:
for element in sequence: block of code
For each element in the sequence the block of code is executed. In the program above, the sequences were generated with the range()
function. range()
creates a list of integers. It's syntax is range([start,] stop[, step])
. The arguments in square brackets are optional. If step
is left out, it defaults to 1
, if start
is left out, it defaults to 0
. It's easy to understand by trying a few examples:
In [24]: range(10) Out[24]: [0, 1, 2, 3, 4, 5, 6, 7, 8, 9] In [25]: range(1,11) Out[25]: [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] In [26]: range(0,25,5) Out[26]: [0, 5, 10, 15, 20]
Exercise 6
Write a function to calculate the sum the series for any n. Allow the user to input the value of n.
The while
statement
A while
statement is used to repeat a block of code until a condition is met. The syntax is:
while condition: block of code
The example below asks the user for a secret password and won't stop until the user enters the the correct password, swordfish
.
passwd = '' # set the initial value of the password to an incorrect result while (passwd != 'swordfish'): passwd = input('What is the secret password: ') print('\nYeah! You know the secret password.')
Defining functions revisited
We saw how to define functions on the Quick Tour page. Now it's time to clean up a few details. Here is the basic syntax for declaring a function:
def function name(arguments): function code
Python functions aren't required required to have an argument or return a result, but most do. Python functions can also have hidden arguments. Here's an example of a Python function with one hidden and one required argument.
In [2]: def hello(name, greeting='Hello'): ...: print('%s %s' % (greeting, name)) ...: print('Have a nice day!') ...: ...: In [3]: hello('bob') Hello bob Have a nice day! In [4]: hello('Bob', greeting='Bonjour') Bonjour Bob Have a nice day!
The first argument, name
, is mandatory. Python will generate an error message if it isn't specified in the call to the function (go ahead and try it). The second argument, greeting
, is optional. It defaults to 'Hello'
if it isn't specified in the function call. You don't have to include the variable assignment in the function call; the syntax hello('Bob','Bonjour') also works. If the variable assignment isn't included, then Python uses the order of the arguments to make the assignment. It's safest to specify the variable names for all optional arguments.
In the example above the function doesn't return any result. The return statement allows you to return a function's output. Here is an example. Download or type it and try it out.
def sumints(): """Return the sum of the first n integers""" sum = 0 for idx in range(1,n+1): sum = sum + idx return sum
Here's a test.
In [25]: run sumints In [26]: sumints(5) Out[26]: 15
The return
statement can return just about any kind of object including lists and numpy arrays.
Modules and Packages
One of Python's greatest strengths is its ability to import modules and packages of code written by others. This strength is derived from the fact that Python is open source software. That means anyone can develop and modify software for Python. Many programers and scientists have contributed to open-source scientific modules and packages for Python. A module is just a collection of functions and data that can be imported into any Python session. A package is essentially a collection of modules.
Options for importing modules
You are already familiar with one way to import a module. On the Quick Tour page we used the command from pylab import *
to import the entire PyLab package. One problem with this approach is that you import everything in the package, even though you may only call a single function from the package in your program. You can avoid this by typing only the functions you want instead of using the wildcard symbol '*'
. For example:
In [2]: from pylab import sin,cos In [3]: sin(3) Out[3]: 0.14112000805986721 In [4]: cos(3) Out[4]: -0.98999249660044542 In [5]: tan(3) --------------------------------------------------------------------------- NameError Traceback (most recent call last) /Users/sburns/<ipython console> in <module>() NameError: name 'tan' is not defined In [6]:
In this example we imported the sin()
and cos()
functions, but not the tan()
function. In many cases using the wildcard to import everything isn't a problem, but suppose you had defined your own function and then imported a module that had a function with the same name. The names would conflict. Python takes the most recent definition of the function and overwrites the previous one. Here is some code that illustrates the problem.
In [1]: from pylab import * In [2]: print(e) 2.71828182846 In [3]: from scipy.constants import * In [4]: print(e) 1.60217653e-19
In this example we import PyLab which defines e = 2.718
, the base of the natural logarithm. When scipy.constants
module is imported it overwrites e
and sets it equal to the charge of the electron, 1.602 × 10-19 C. We can avoid this problem by importing scipy.constants
under another name by using the command import scipy.constants as pc
. We can access the charge of electron by typing pc.e
.
In [1]: from pylab import * In [2]: import scipy.constants as pc In [3]: print(e) 2.71828182846 In [4]: print(pc.e) 1.60217653e-19
In this case we imported all of the PyLab packages with the wildcard and imported the scipy.constants
module under the alias pc
. We will follow this convention in all future Toolbox programs and functions to avoid any conflicts between the two modules.
Writing your own modules
Writing your own modules is trivial. You just enter the Python code you want to be included in the module into a file (name the file with the .py
extension of course) and import it with the same import
commands discussed above. The only thing to keep in mind is that you must specify the path to your modules. If your module is in your current working directory, just enter the file name of the module without the .py
extension. The module mypolar.py
shown below contains two functions. One converts cartesian to polar coordinates, the other converts polar to x-y coordinates.
""" This is a module contains functions to convert to and from cartesian to cylindrical polar coordinates. """ # The functions below need these sqrt and trig functions, so lets import them. from pylab import sqrt,arctan,cos,sin def xy2rth(x, y): """Converts x and y coordinates to polar coordinates, r and theta.""" r = sqrt(x**2 + y**2) theta = arctan(y/x) return [r, theta] def rth2xy(r,theta): """Converts the polar coordinates r and theta to x and y.""" x = r*cos(theta) y = r*sin(theta) return [x, y]
Let's test it.
In [1]: from mypolar import * In [2]: r,theta = xy2rth(1,1) In [3]: print(r,theta) (1.4142135623730951, 0.78539816339744828) In [4]: xnew, ynew = rth2xy(r,theta) In [5]: print(xnew,ynew) (1.0000000000000002, 1.0)
It seems to work.
Exercise 7
Can you find some deficiencies in these functions? Try the x-y coordinates (-1, -1). Convert to polar coordinates then convert those polar coordinates back to cartesian coordinates. Do you get back the same x-y coordinates? Why not? Edit mypolar.py
so that it gives the correct result. You might find the PyLab function arctan2(y,x)
useful. It returns arctan(y/x), in radians. The result is between -π and π. The vector in the plane from the origin to point (x, y) makes this angle with the positive x axis. The function arctan2()
works because the signs of both inputs are known to it, so it can compute the correct quadrant for the angle. Remember that after you edit the module you will have to import it in a new IPython session to force Python to recognize the changes.
Using IPython help()
The IPython command help('module_name')
will print information about the module as well as the docstring for the module and all the functions it contains. This command will also print the information and the docstring for any script in your current directory. The command help(function_name)
, without the quotes, will print the docstring for any function that has been imported. Typing help()
with no arguments runs IPython's interactive help program. Type help('help')
to learn how to use it. Try out the help
command on the pylab
module, the plot
command in pylab
(don't forget to import pylab
first), and the mypolar
module.